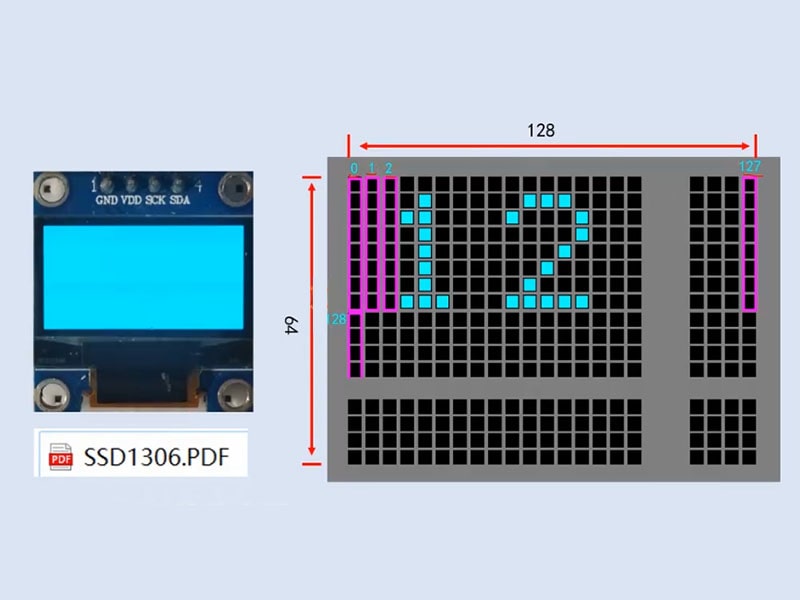
Preface
This article will take you through how to write a driver for an OLED display. Here I am using hardware IIC from HAL library and OLED screen from SSD1306.Here you need to refer to the datasheet of SSD1306 to write the driver.
Manual location:
1. Device address of OLED
The device address of the OLED consists of 8 bits, which are 011110SA0 R/W#.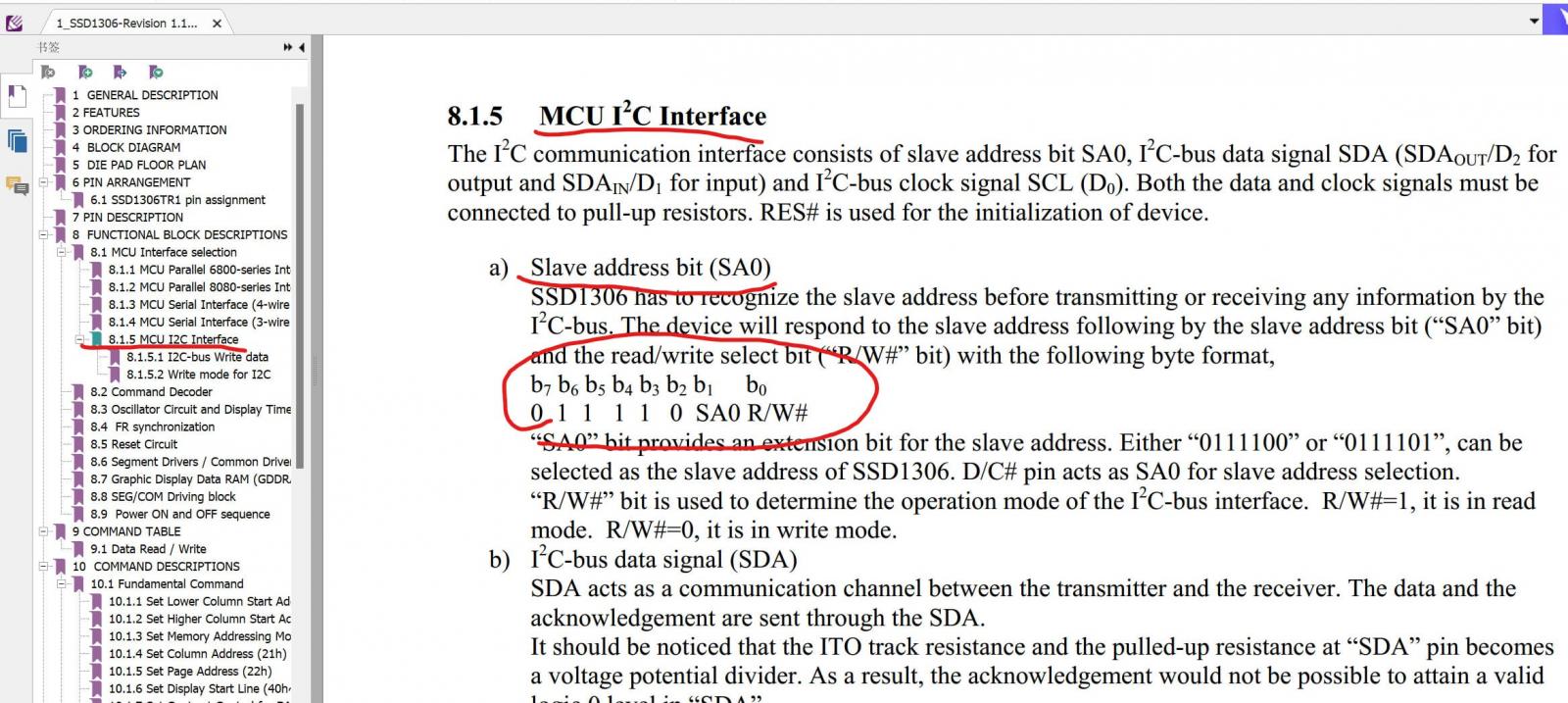
The datasheet has this paragraph:
D/C# pin acts as SA0 for slave address selection
According to the datasheet, D/C# pin acts as SA0 for slave address selection.
According to the OLED schematic we can see that D/C is connected to GND so SA0 = 0.
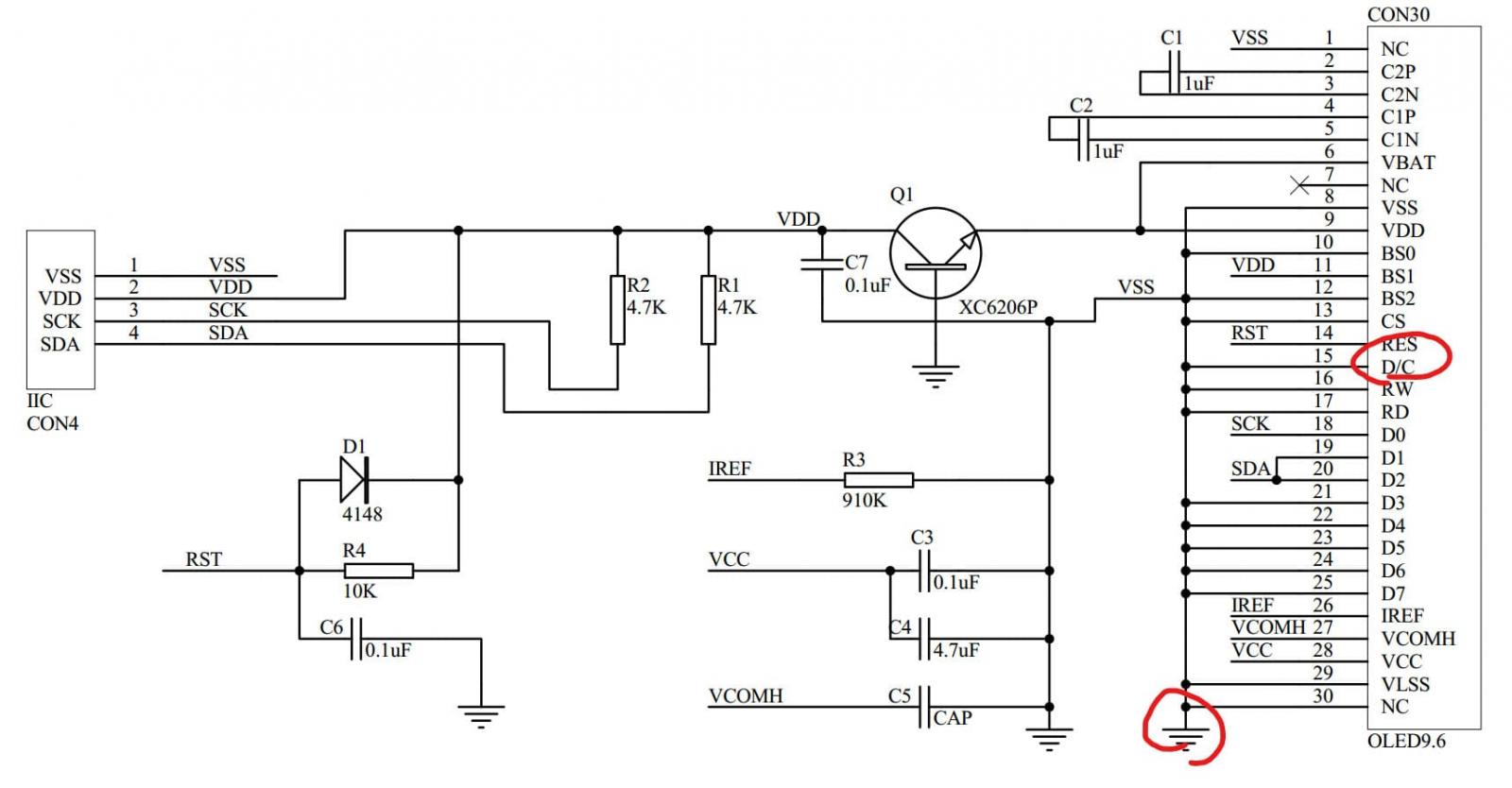
R/W bit: this bit is 0 when data is to be written and 1 when data is to be read.
So the device address of the OLED can be derived:
Write address: 0x78
Read address: 0x79
2. Write data and write command function
Write Command FunctionThe HAL_I2C_Mem_Write function that comes with the HAL library is called here to write commands and data.
We need to refer to the I2C-bus Write data section in the datasheet to write the function.
According to the datasheet:
The first step sends the device address first (here it is write data which is 0x78).
The second step is to send the control byte:
The control byte is determined by the Co bit and the D/C bit.
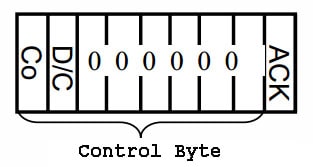
D/C is 0 and Co is 0 when a command is being sent.
When data is sent, D/C is 1 and Co is 0.
So the control byte is 0x00 when sending command and 0x40 when sending data.
After the transmission of the slave address,either the control byte or the data byte may be sent acrossthe SDA.A control byte mainly consists of Co and D/C#bits following by six“0"‘s.
a.If the Co bit is set as logic“0”,the transmission of the following information will containdata bytes only.
b.The D/C#bit determines the next data byte is acted as a command or a data.If the D/C#bit is set to logic O,it defmes the following data byte as a command.If the D1C#bit is sertologic“1”,it defines the following data byte as a data which will be stored at the GDDRAM.The GDDRAM column address pointer will be increased by one automatically after each data write.
a.If the Co bit is set as logic“0”,the transmission of the following information will containdata bytes only.
b.The D/C#bit determines the next data byte is acted as a command or a data.If the D/C#bit is set to logic O,it defmes the following data byte as a command.If the D1C#bit is sertologic“1”,it defines the following data byte as a data which will be stored at the GDDRAM.The GDDRAM column address pointer will be increased by one automatically after each data write.
The third step sends the data bytes which are the parameters uint8_t data and uint8_t cmd in the following function.
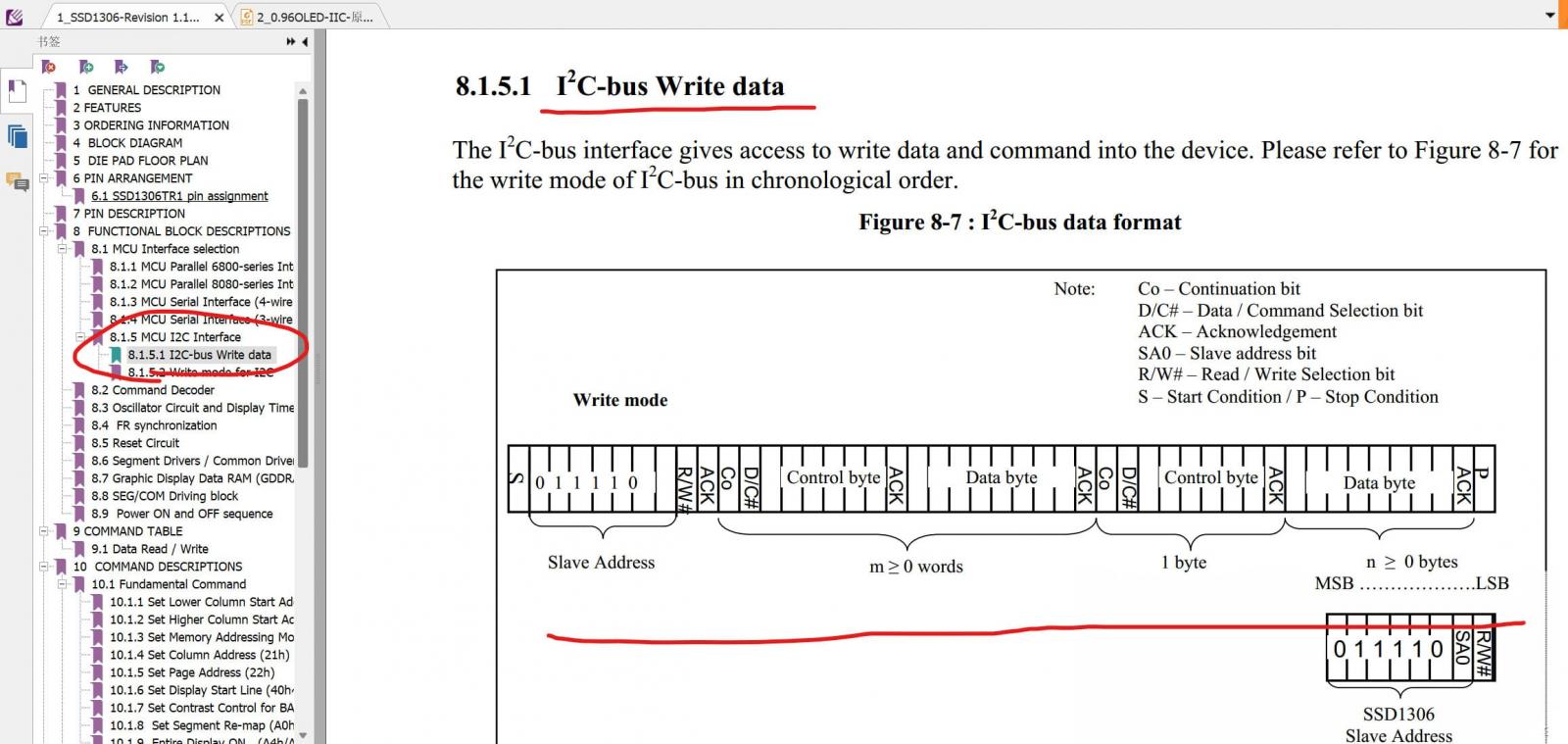
1丨void OLED_WR_CMD(uint8_t cmd)
2丨{
3丨HAL_I2C_Mem_Write(&hi2c1, 0x78, 0x00, I2C_MEMADD_SIZE_8BIT, &cmd, 1, 0x100);
4丨}
2丨{
3丨HAL_I2C_Mem_Write(&hi2c1, 0x78, 0x00, I2C_MEMADD_SIZE_8BIT, &cmd, 1, 0x100);
4丨}
Write Data Functions
1void OLED_WR_DATA(uint8_t data)
2{
3HAL_I2C_Mem_Write(&hi2c1, 0x78, 0x40, I2C_MEMADD_SIZE_8BIT, &data, 1, 0x100);
4}
2{
3HAL_I2C_Mem_Write(&hi2c1, 0x78, 0x40, I2C_MEMADD_SIZE_8BIT, &data, 1, 0x100);
4}
3. write the initialization OLED screen function
The following are the steps to initialize the OLED display.
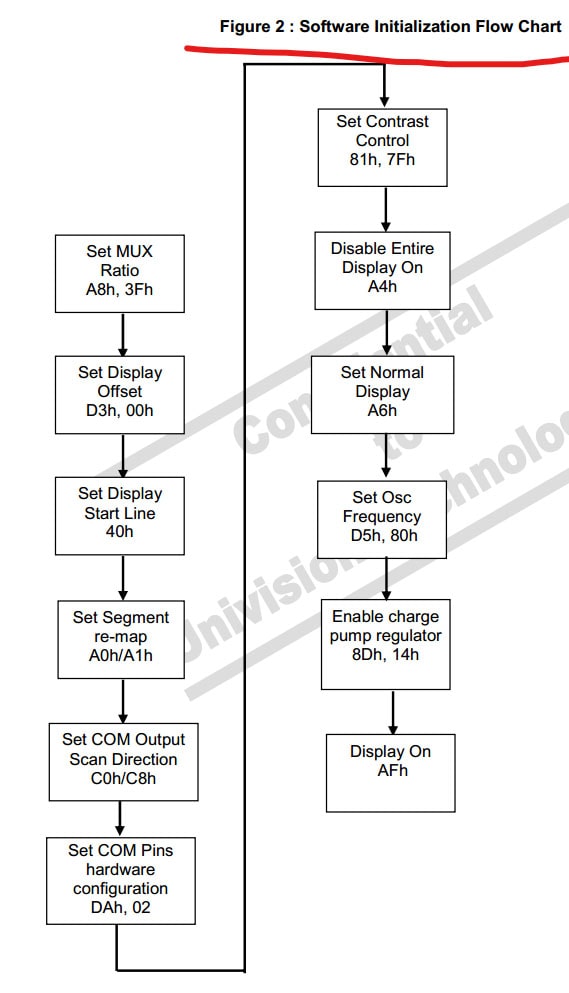
These parameters to be set are placed in the CMD_Data array
1丨uint8_t CMD_Data[] = {
2丨0xAE, 0x00, 0x10, 0x40, 0xB0, 0x81, 0xFF, 0xA1, 0xA6, 0xA8, 0x3F,
3丨
4丨0xC8, 0xD3, 0x00, 0xD5, 0x80, 0xD8, 0x05, 0xD9, 0xF1, 0xDA, 0x12,
5丨
6丨0xD8, 0x30, 0x8D, 0x14, 0xAF }; //INITIAL COMMAND
2丨0xAE, 0x00, 0x10, 0x40, 0xB0, 0x81, 0xFF, 0xA1, 0xA6, 0xA8, 0x3F,
3丨
4丨0xC8, 0xD3, 0x00, 0xD5, 0x80, 0xD8, 0x05, 0xD9, 0xF1, 0xDA, 0x12,
5丨
6丨0xD8, 0x30, 0x8D, 0x14, 0xAF }; //INITIAL COMMAND
Here a for loop is used to write the parameters in
1丨void WriteCmd(void)
2丨{
3丨 uint8_t i = 0;
4丨 for (i = 0; i < 27; i++)
5丨 {
6丨 HAL_I2C_Mem_Write(&hi2c1, 0x78, 0x00, I2C_MEMADD_SIZE_8BIT, CMD_Data + i, 1, 0x100);
7丨 }
8丨}
9丨
10丨//initialize oled screen
11丨void OLED_Init(void)
12丨{
13丨 HAL_Delay(200);
14丨
15丨 WriteCmd();
16丨}
2丨{
3丨 uint8_t i = 0;
4丨 for (i = 0; i < 27; i++)
5丨 {
6丨 HAL_I2C_Mem_Write(&hi2c1, 0x78, 0x00, I2C_MEMADD_SIZE_8BIT, CMD_Data + i, 1, 0x100);
7丨 }
8丨}
9丨
10丨//initialize oled screen
11丨void OLED_Init(void)
12丨{
13丨 HAL_Delay(200);
14丨
15丨 WriteCmd();
16丨}
4.Other functions
Clear the screen function1丨//Clear Screen
2丨void OLED_Clear(void)
3丨{
4丨 uint8_t i, n;
5丨 for (i = 0; i < 8; i++)
6丨 {
7丨 OLED_WR_CMD(0xb0 + i);
8丨 OLED_WR_CMD(0x00);
9丨 OLED_WR_CMD(0x10);
10丨 for (n = 0; n < 128; n++)
11丨 OLED_WR_DATA(0);
12丨 }
13丨}
2丨void OLED_Clear(void)
3丨{
4丨 uint8_t i, n;
5丨 for (i = 0; i < 8; i++)
6丨 {
7丨 OLED_WR_CMD(0xb0 + i);
8丨 OLED_WR_CMD(0x00);
9丨 OLED_WR_CMD(0x10);
10丨 for (n = 0; n < 128; n++)
11丨 OLED_WR_DATA(0);
12丨 }
13丨}
Turning the OLED display on and off Functions for OLED display
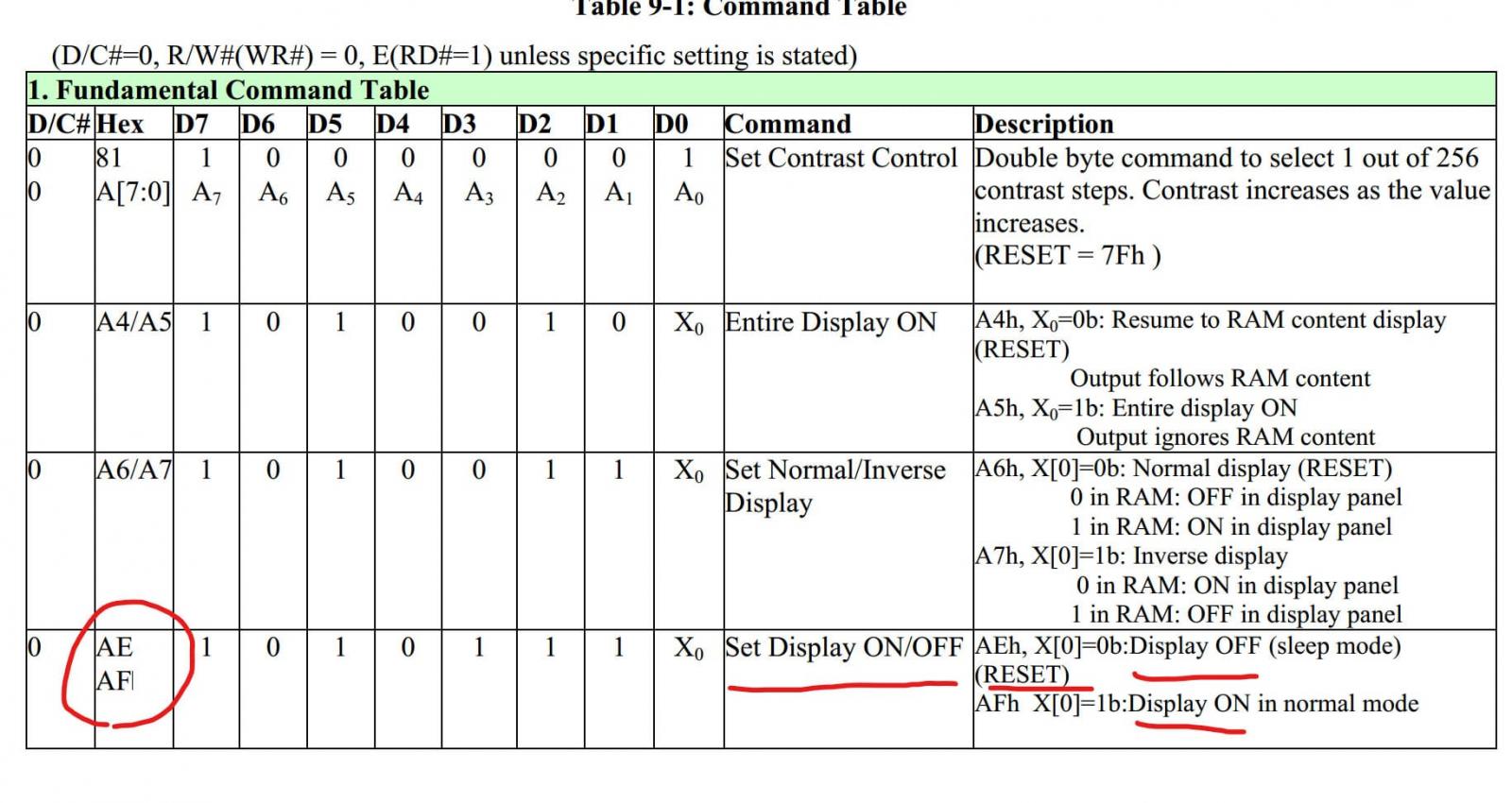
1丨//Turn on OLED Display
2丨void OLED_Display_On(void)
3丨{
4丨 OLED_WR_CMD(0X8D); //SET DCDC command
5丨 OLED_WR_CMD(0X14); //DCDC ON
6丨 OLED_WR_CMD(0XAF); //DISPLAY ON
7丨}
8丨//Turn off OLED Display
9丨void OLED_Display_Off(void)
10丨{
11丨 OLED_WR_CMD(0X8D); //SET DCDC command
12丨 OLED_WR_CMD(0X10); //DCDC OFF
13丨 OLED_WR_CMD(0XAE); //DISPLAY OFF
14丨}
2丨void OLED_Display_On(void)
3丨{
4丨 OLED_WR_CMD(0X8D); //SET DCDC command
5丨 OLED_WR_CMD(0X14); //DCDC ON
6丨 OLED_WR_CMD(0XAF); //DISPLAY ON
7丨}
8丨//Turn off OLED Display
9丨void OLED_Display_Off(void)
10丨{
11丨 OLED_WR_CMD(0X8D); //SET DCDC command
12丨 OLED_WR_CMD(0X10); //DCDC OFF
13丨 OLED_WR_CMD(0XAE); //DISPLAY OFF
14丨}
Setting the Coordinate Function
Here the OLED is set to page address mode and the address range is 0xb0-0xb7. that is, the range of y is 0-7.
The range of x is 0-127
1丨void OLED_Set_Pos(uint8_t x, uint8_t y)
2丨{
3丨 OLED_WR_CMD(0xb0 + y);
4丨 OLED_WR_CMD(((x & 0xf0) >> 4) | 0x10);
5丨 OLED_WR_CMD(x & 0x0f);
6丨}
2丨{
3丨 OLED_WR_CMD(0xb0 + y);
4丨 OLED_WR_CMD(((x & 0xf0) >> 4) | 0x10);
5丨 OLED_WR_CMD(x & 0x0f);
6丨}
5.Display of characters and numeric function writing
1丨//Display 2 numbers
2丨//x,y : Origin coordinate
3丨//len : Number of digits
4丨//size: Font size
5丨//mode: 0, Fill mode; 1, Superposition mode
6丨//num: (0~4294967295);
7丨void OLED_ShowNum(uint8_t x, uint8_t y, unsigned int num, uint8_t len, uint8_t size2)
8丨{
9丨 uint8_t t, temp;
10丨 uint8_t enshow = 0;
11丨 for (t = 0; t < len; t++)
12丨 {
13丨 temp = (num / oled_pow(10, len - t - 1)) % 10;
14丨 if (enshow == 0 && t < (len - 1))
15丨 {
16丨 if (temp == 0)
17丨 {
18丨 OLED_ShowChar(x + (size2 / 2)*t, y, ' ', size2);
19丨 continue;
20丨 }
21丨 else enshow = 1;
22丨
23丨 }
24丨 OLED_ShowChar(x + (size2 / 2)*t, y, temp + '0', size2);
25丨 }
26丨}
27丨//Displays a character, including partial characters, at a specified position
28丨//x:0~127
29丨//y:0~63
30丨//mode: 0,Anti-white display; 1. It is displayed normally
31丨//size: Select font16/12
32丨void OLED_ShowChar(uint8_t x, uint8_t y, uint8_t chr, uint8_t Char_Size)
33丨{
34丨 unsigned char c = 0, i = 0;
35丨 c = chr - ' ';// The offset value is obtained
36丨 if (x > 128 - 1) { x = 0; y = y + 2; }
37丨 if (Char_Size == 16)
38丨 {
39丨 OLED_Set_Pos(x, y);
40丨 for (i = 0; i < 8; i++)
41丨 OLED_WR_DATA(F8X16[c * 16 + i]);
42丨 OLED_Set_Pos(x, y + 1);
43丨 for (i = 0; i < 8; i++)
44丨 OLED_WR_DATA(F8X16[c * 16 + i + 8]);
45丨 }
46丨 else {
47丨 OLED_Set_Pos(x, y);
48丨 for (i = 0; i < 6; i++)
49丨 OLED_WR_DATA(F6x8[c][i]);
50丨
51丨 }
52丨}
53丨
54丨// Displays a string of character numbers
55丨void OLED_ShowString(uint8_t x, uint8_t y, uint8_t *chr, uint8_t Char_Size)
56丨{
57丨 unsigned char j = 0;
58丨 while (chr[j] != '
2丨//x,y : Origin coordinate
3丨//len : Number of digits
4丨//size: Font size
5丨//mode: 0, Fill mode; 1, Superposition mode
6丨//num: (0~4294967295);
7丨void OLED_ShowNum(uint8_t x, uint8_t y, unsigned int num, uint8_t len, uint8_t size2)
8丨{
9丨 uint8_t t, temp;
10丨 uint8_t enshow = 0;
11丨 for (t = 0; t < len; t++)
12丨 {
13丨 temp = (num / oled_pow(10, len - t - 1)) % 10;
14丨 if (enshow == 0 && t < (len - 1))
15丨 {
16丨 if (temp == 0)
17丨 {
18丨 OLED_ShowChar(x + (size2 / 2)*t, y, ' ', size2);
19丨 continue;
20丨 }
21丨 else enshow = 1;
22丨
23丨 }
24丨 OLED_ShowChar(x + (size2 / 2)*t, y, temp + '0', size2);
25丨 }
26丨}
27丨//Displays a character, including partial characters, at a specified position
28丨//x:0~127
29丨//y:0~63
30丨//mode: 0,Anti-white display; 1. It is displayed normally
31丨//size: Select font16/12
32丨void OLED_ShowChar(uint8_t x, uint8_t y, uint8_t chr, uint8_t Char_Size)
33丨{
34丨 unsigned char c = 0, i = 0;
35丨 c = chr - ' ';// The offset value is obtained
36丨 if (x > 128 - 1) { x = 0; y = y + 2; }
37丨 if (Char_Size == 16)
38丨 {
39丨 OLED_Set_Pos(x, y);
40丨 for (i = 0; i < 8; i++)
41丨 OLED_WR_DATA(F8X16[c * 16 + i]);
42丨 OLED_Set_Pos(x, y + 1);
43丨 for (i = 0; i < 8; i++)
44丨 OLED_WR_DATA(F8X16[c * 16 + i + 8]);
45丨 }
46丨 else {
47丨 OLED_Set_Pos(x, y);
48丨 for (i = 0; i < 6; i++)
49丨 OLED_WR_DATA(F6x8[c][i]);
50丨
51丨 }
52丨}
53丨
54丨// Displays a string of character numbers
55丨void OLED_ShowString(uint8_t x, uint8_t y, uint8_t *chr, uint8_t Char_Size)
56丨{
57丨 unsigned char j = 0;
58丨 while (chr[j] != '